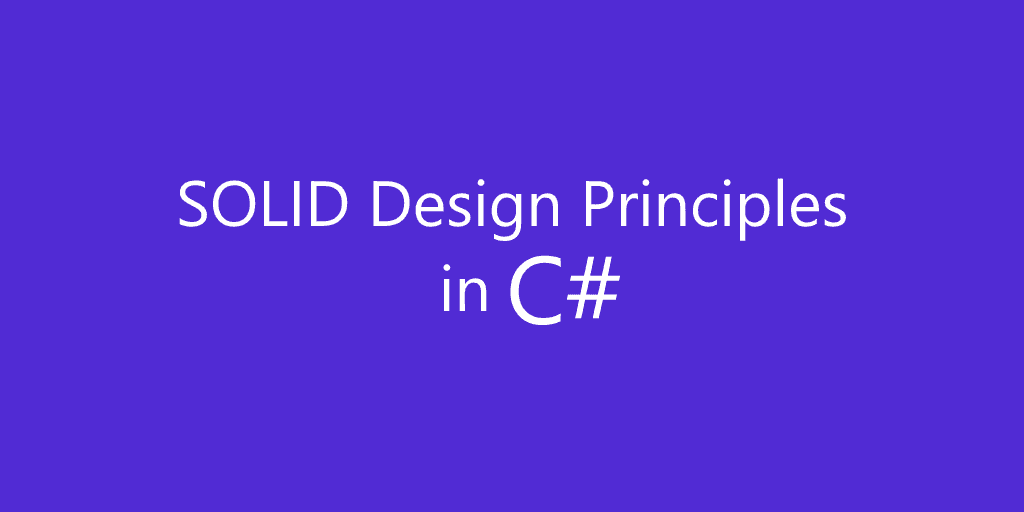
SOLID Design Principles in C# - Part 4 - Interface Segregation
The Interface Segregation Principle (ISP) is a principle that helps us to think about creating many specialized interfaces instead of a single general-purpose interface.
This post is part of a series where we explore the SOLID design principles, arguably the most popular design principles for object-oriented software development.
Interface Segregation Principle
Make fine grained interfaces that are client specific. Robert C. Martin
Interfaces should be cohesive, clients should never be forced to implement an interface that they don't use, or clients shouldn't be forced to depend on methods they do not use.
When interfaces aggregate lots of generic behaviours they become difficult to maintain and evolve. The rule of thumb is "the more specific the better".
If you think about the (Single-Responsibility Principle (SRP))1, the same principles are applied here.
The wrong example
The same example as SRP, but this time we represent it through the use of an interface, a fat one:
public interface IOrderProcessor
{
void Validate();
void Save();
void Notify();
}
In this case, we only want to validate an order, but because we have a single general-purpose interface, the client is mandated to implement methods that aren't needed:
public class OrderValidator : IOrderProcessor
{
private readonly Order _order;
public OrderValidator(Order order)
{
_order = order;
}
// Validate
public void Validate()
{
//TODO: validate order
Console.WriteLine($"validate order: {_order.OrderNumber}");
}
// Save
public void Save()
{
throw new NotImplementedException();
}
// Notify
public void Notify()
{
throw new NotImplementedException();
}
}
In general, you get to see NotImplementedException
when the principle is violated.
The right example
The more specific the better:
public interface IOrderValidator
{
void Validate();
}
Similar to the right example from SRP, this one uses the specific interface IOrderSaver
:
public class OrderSaver : IOrderSaver
{
private readonly Order _order;
public OrderSaver(Order order)
{
_order = order;
}
public void Save()
{
//TODO: save order
Console.WriteLine($"save order: {_order.OrderNumber}");
}
}
I invite you to have a look at the repository and run the examples:
The complete Interface Segregation principle example is available on PlayGoKids repository.
Full Series
Single-Responsibility Principle (SRP)
Liskov Substitution Principle (LSP)
Interface Segregation Principle (ISP)