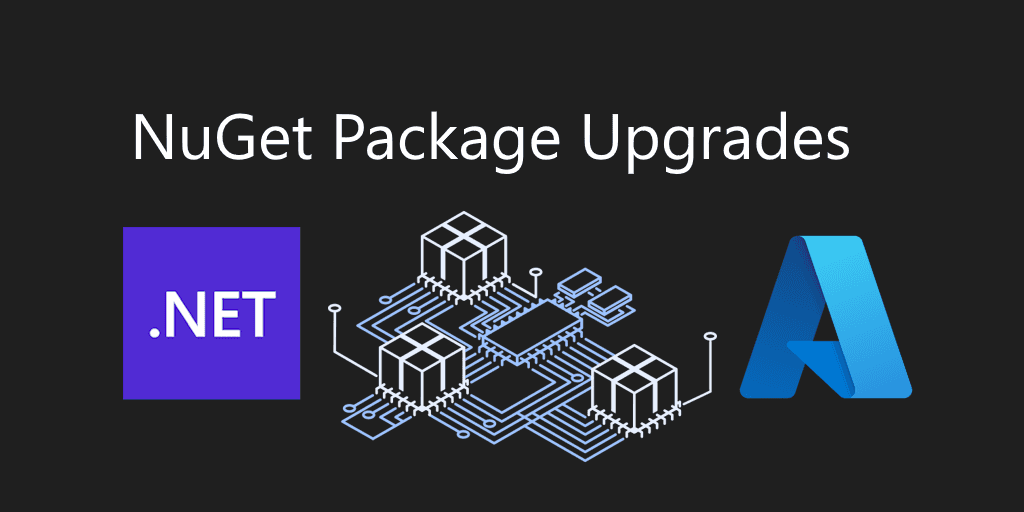
NuGet Package Upgrade - WindowsAzure.Storage To Azure.Storage.Blobs
Microsoft deprecated the WindowsAzure.Storage
library in favor of Azure.Storage.*
libraries, which offers a modernized API with better performance, security, and maintainability. However, migrating from the older package to the newer ones can present challenges due to significant API differences and breaking changes.
This article explores these challenges and provides a step-by-step approach to migrating blob storage
operations.
Key Differences Between Packages
Deprecated package
<PackageReference Include="WindowsAzure.Storage" Version="9.3.3" />
New package
<PackageReference Include="Azure.Storage.Blobs" Version="12.24.0" />
The new SDK introduces a more efficient design based on modern .NET principles. Some of the major differences include:
- No direct equivalent for
CloudBlockBlob
,CloudPageBlob
, orCloudBlobDirectory
- Different approach to listing blobs (hierarchical listing vs. flat listing)
- New async paging methods for blob enumeration
- Updated authentication mechanisms
Migration Example: Listing Blobs in a Container
Below, I am comparing the old implementation with the new one.
Old Implementation (WindowsAzure.Storage)
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Blob;
/// <summary>
/// Lists blobs in containers
/// </summary>
/// <returns></returns>
public async Task<string> ListContainerBlobs()
{
StringBuilder builder = new StringBuilder();
BlobContinuationToken token = null;
do
{
BlobResultSegment resultSegment = await _unmoderatedContainer.ListBlobsSegmentedAsync(token);
token = resultSegment.ContinuationToken;
foreach (IListBlobItem item in resultSegment.Results)
{
if (item.GetType() == typeof(CloudBlockBlob))
{
CloudBlockBlob blob = (CloudBlockBlob)item;
builder.AppendLine($"Block blob of length {blob.Properties.Length}: {blob.Uri}");
}
else if (item.GetType() == typeof(CloudPageBlob))
{
CloudPageBlob pageBlob = (CloudPageBlob)item;
builder.AppendLine($"Page blob of length {pageBlob.Properties.Length}: {pageBlob.Uri}");
}
else if (item.GetType() == typeof(CloudBlobDirectory))
{
CloudBlobDirectory directory = (CloudBlobDirectory)item;
builder.AppendLine($"Directory: {directory.Uri}");
}
}
} while (token != null);
return builder.ToString();
}
New Implementation (Azure.Storage.Blobs)
using Azure.Storage.Blobs;
/// <summary>
/// Lists blobs in containers
/// </summary>
/// <returns></returns>
public async Task<string> ListContainerBlobs()
{
StringBuilder builder = new StringBuilder();
string containerUri = _unmoderatedContainer.Uri.ToString();
string continuationToken = null;
do
{
var resultSegment = _unmoderatedContainer.GetBlobsByHierarchyAsync(delimiter: "/").AsPages(continuationToken);
await foreach (var blobPage in resultSegment)
{
continuationToken = blobPage.ContinuationToken;
foreach (var item in blobPage.Values)
{
if (item.IsBlob)
{
var blobItem = item.Blob;
var blobUri = $"{containerUri}/{blobItem.Name}";
builder.AppendLine($"Block blob of length {blobItem.Properties.ContentLength}: {blobUri}");
}
else if (item.IsPrefix)
{
var prefixItem = item.Prefix;
builder.AppendLine($"Directory: {containerUri}/{prefixItem}");
}
}
}
} while (continuationToken != null);
return builder.ToString();
}
Challenges and How to Overcome Them
CloudBlockBlob
andCloudPageBlob
Are No Longer Available
- In the new SDK,
BlobItem
represents blobs, andBlobProperties
stores metadata. - The
IsBlob
property helps differentiate between blobs and directories.
- No
CloudBlobDirectory
Equivalent
- Instead of
CloudBlobDirectory
, the new SDK usesPrefix
to represent virtual directories.
- Continuation Tokens Work Differently
- In
WindowsAzure.Storage
, pagination usesBlobContinuationToken
. - In
Azure.Storage.Blobs
, pagination is done viaAsPages()
with a continuation token string.
- Different Methods for Listing Blobs
ListBlobsSegmentedAsync()
is replaced byGetBlobsByHierarchyAsync().AsPages()
.- The new approach allows for hierarchical navigation using delimiters.
Conclusion
Migrating from WindowsAzure.Storage
to Azure.Storage.Blobs
requires adapting to a more modern, efficient API. The transition is not always straightforward, as there are significant differences in class structures, methods, and pagination mechanisms. A direct conversion is not possible in many cases, requiring us to rethink the implementation.
While these changes can be challenging, they ultimately lead to better performance, improved security, and more maintainable code. Try understanding these differences and planning the migration carefully.